티스토리 뷰
* 인프런에 있는 "홍정모의 게임 만들기 연습 문제 패키지" 강의를 바탕으로 작성된 글입니다.
1.움직이는 마우스 커서 위치에 회전하는 별 그려보기
namespace shyplants
{
class MouseExample : public Game2D
{
private:
float time = 0.0f;
public:
void update() override
{
const vec2 mouse_pos = getCursorPos();
translate(mouse_pos); // 실행순서 3. 마우스 위치로 제자리 회전중인 별이 이동한다.
rotate(time * 60.0f); // 실행순서 2. 제자리에서 별이 회전한다.
drawFilledStar(Colors::gold, 0.2f, 0.1f); // 실행순서 1. 원점에 별을 그린다.
time += getTimeStep();
}
};
}
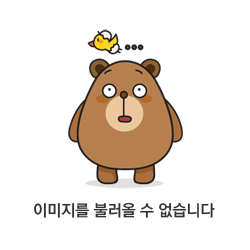
2. 파란 원을 하나 그리고 마우스가 그 원에 들어갔을 경우 빨간색으로 변경하기
namespace shyplants
{
class MouseExample : public Game2D
{
private:
float time = 0.0f;
float radius = 0.3f; // 원의 반지름
public:
void update() override
{
const vec2 mouse_pos = getCursorPos();
if (mouse_pos.x*mouse_pos.x + mouse_pos.y*mouse_pos.y <= radius * radius)
{
drawFilledCircle(Colors::red, radius); // 마우스 좌표(x,y)가 원 안에 위치할 때 빨간색 원 그리기
}
else
{
drawFilledCircle(Colors::blue, radius); // 마우스 좌표(x,y)가 원 안에 위치하지 않을시 파랑색 원 그리기
}
time += getTimeStep();
}
};
}
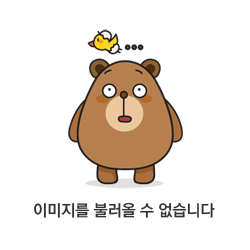
3. 화면 가운데 물체를 커서방향으로 회전하기 & 마우스 버튼을 누르면 커서 방향으로 총알을 발사하기
namespace shyplants
{
class MyBullet
{
public:
vec2 center = vec2(0.0f, 0.0f);
vec2 velocity = vec2(0.0f, 0.0f);
void draw()
{
beginTransformation();
translate(center);
drawFilledRegularConvexPolygon(Colors::yellow, 0.02f, 8);
drawWiredRegularConvexPolygon(Colors::gray, 0.02f, 8);
endTransformation();
}
void update(const float& dt)
{
center += velocity * dt;
}
};
class MouseExample : public Game2D
{
private:
float time = 0.0f;
float radius = 0.1f;
float angle, rad;
std::list<MyBullet*> bulletList;
public:
bool OOB(vec2& pos)
{
return pos.y < -1.0f || pos.y > 1.0f || pos.x < -1.0f || pos.x > 1.0f;
}
void update() override
{
const vec2 mouse_pos = getCursorPos(true);
beginTransformation();
{
if (mouse_pos.x != 0.0f) {
rad = atan2f(mouse_pos.y, mouse_pos.x);
angle = 180.0f / 3.141592f * rad;
rotate(angle);
}
translate(vec2(0.1f, 0));
drawFilledBox(Colors::blue, 0.2f, 0.05f);
}
endTransformation();
drawFilledCircle(Colors::blue, radius);
if (isMouseButtonPressedAndReleased(GLFW_MOUSE_BUTTON_1)) // 왼쪽 마우스버튼 클릭시 마우스 좌표 각도에 따른 총알 발사
{
if (mouse_pos.x != 0.0f)
{
bulletList.push_back(new MyBullet);
bulletList.back()->center = vec2(0, 0);
bulletList.back()->center.x += (radius + 0.1f) * cos(rad);
bulletList.back()->center.y += (radius + 0.1f) * sin(rad);
bulletList.back()->velocity = vec2(2.0f*cos(rad), 2.0f*sin(rad));
}
}
// 총알 리스트를 순회하며 업데이트, 드로우 함수 호출
for (auto iter = bulletList.begin(); iter != bulletList.end(); iter++)
{
(*iter)->update(getTimeStep());
(*iter)->draw();
}
// 총알 리스트를 순회하며 화면 밖에 나간 총알 메모리 해제 (메모리 누수 방지)
for (auto iter = bulletList.begin(); iter != bulletList.end();)
{
if (OOB((*iter)->center)) {
delete *iter;
iter = bulletList.erase(iter);
}
else {
iter++;
}
}
time += getTimeStep();
}
};
}
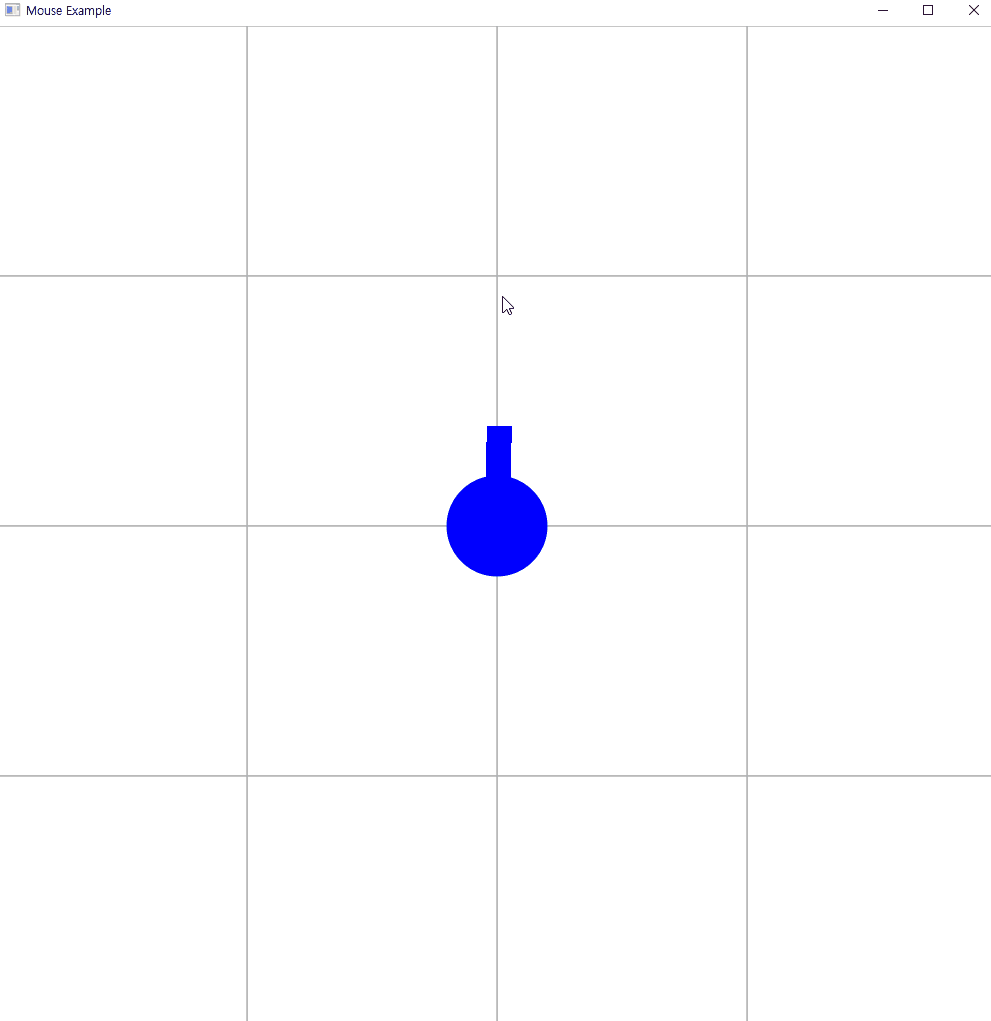
'개발 > 게임개발' 카테고리의 다른 글
c++ 게임만들기 연습문제 2.2 (0) | 2023.02.27 |
---|---|
c++ 게임만들기 연습문제 2.1 (0) | 2023.02.27 |
c++ 게임만들기 연습문제 1.5 (1) | 2023.02.01 |
c++ 게임만들기 연습문제 1.3 (0) | 2023.01.31 |
c++ 게임만들기 연습문제 1.2 (0) | 2023.01.31 |
공지사항
최근에 올라온 글
최근에 달린 댓글
- Total
- Today
- Yesterday
링크
TAG
- 정보올림피아드
- BOJ 27469
- C++게임개발
- Codeforces
- UE5.3
- DP
- 코드포스
- 퀸 움직이기
- 백준 27469
- ndisplay
- 백준 2365
- 언리얼 자동화
- OpenVDB
- pygame
- BOJ 2365
- tetris
- Python
- opengl
- 언리얼 프로젝트 재생성
- ICPC 후기
- 브레젠험 알고리즘
- 초등부
- C++게임
- 홍정모의 게임 만들기 연습 문제 패키지
- unreal enigne
- 숫자판 만들기
- 언리얼 프로젝트 재생성 자동화
- 백준
- 테트리스
- Unreal Engine
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
글 보관함